The quickest way to integrate Sentry.io with NestJS framework without using Raven
First of all, install the required Sentry Node SDK with npm or yarn:
# Using yarn
$ yarn add @sentry/node@5.9.0# Or use npm
$ npm install @sentry/node@5.9.0
Locate your Sentry DSN by going to the project settings:
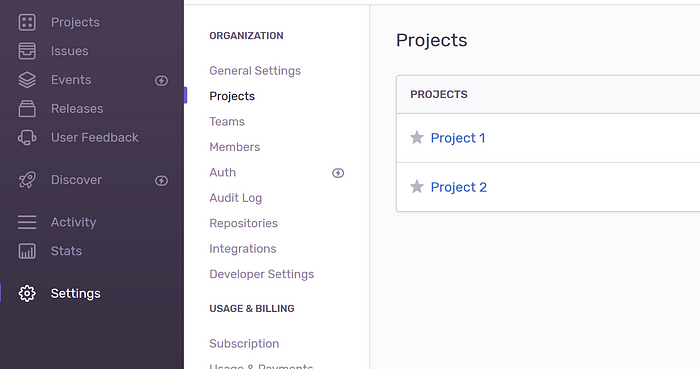
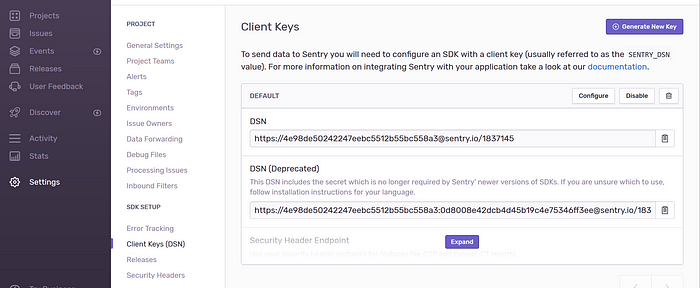
Add these lines to src/main.ts file and change it to your own DSN:
import * as Sentry from '@sentry/node';Sentry.init({
dsn: 'https://4e98de50242247eebc5512b55bc558a3@sentry.io/1837145',
});
An example for the implementation would be like this:
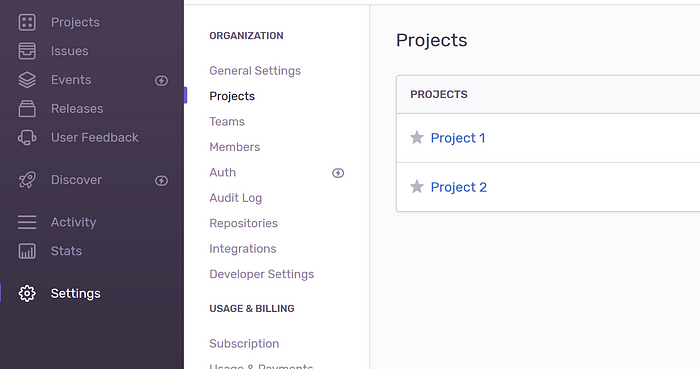
Create a new interceptor file:
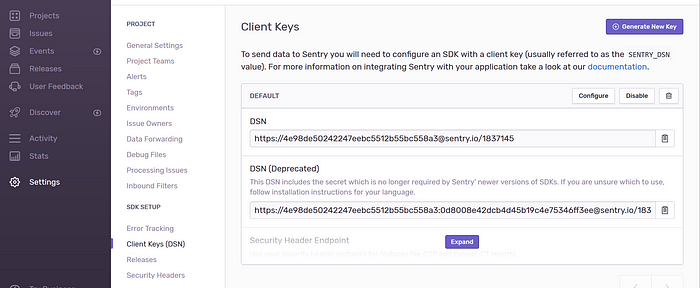
Inject that interceptor to the desired controller by adding these lines:
import { UseInterceptors } from '@nestjs/common';
import { SentryInterceptor } from './sentry.interceptor';@UseInterceptors(SentryInterceptor)
An example on how those codes were to be added:
import { NestFactory } from '@nestjs/core'; | |
import { AppModule } from './app.module'; | |
import * as Sentry from '@sentry/node'; | |
async function bootstrap() { | |
const app = await NestFactory.create(AppModule); | |
Sentry.init({ | |
dsn: 'https://4e98de50242247eebc5512b55bc558a3@sentry.io/1837145', | |
}); | |
await app.listen(3000); | |
} | |
bootstrap(); |
And that’s it!
To test if it’s working, you can add an error exception to a route and make requests to that route. For example:
import { | |
ExecutionContext, | |
Injectable, | |
NestInterceptor, | |
CallHandler, | |
} from '@nestjs/common'; | |
import { Observable } from 'rxjs'; | |
import { tap } from 'rxjs/operators'; | |
import * as Sentry from '@sentry/minimal'; | |
@Injectable() | |
export class SentryInterceptor implements NestInterceptor { | |
intercept( context: ExecutionContext, next: CallHandler): Observable<any> { | |
return next | |
.handle() | |
.pipe( | |
tap(null, (exception) => { | |
Sentry.captureException(exception); | |
}), | |
); | |
} | |
} |
Then check if it’s recorded in your Sentry’s project dashboard:
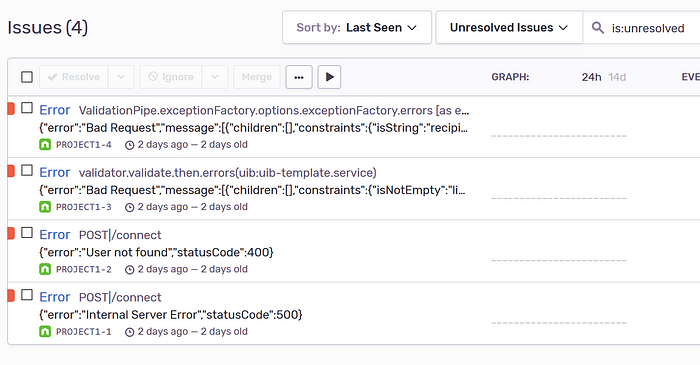